Google Analytics 4 (GA4) Ecommerce Event Tracking on Shopify
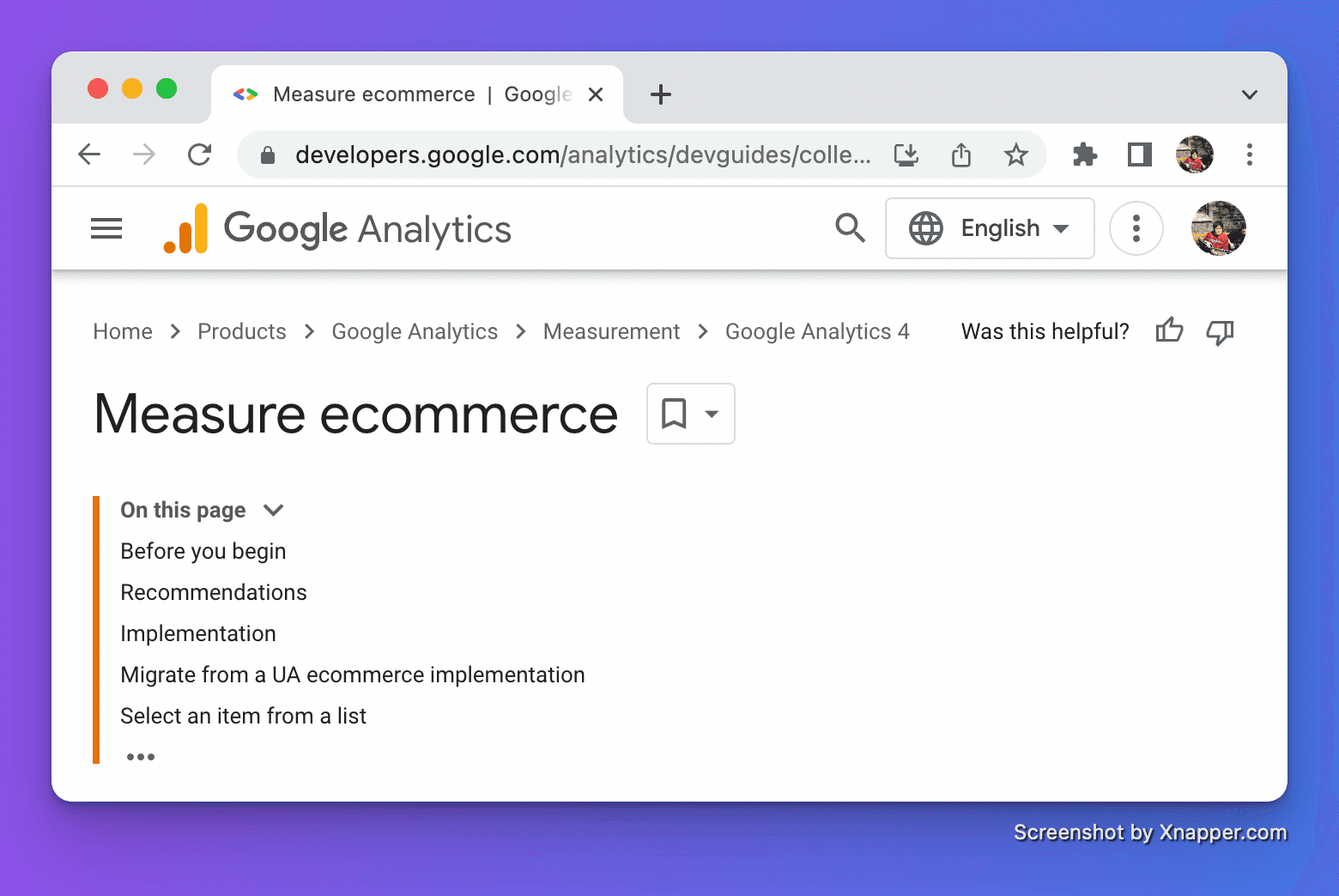
Recently I recevied a Google Analytics 4 tag from a client, that I dutifully pasted into their theme as per usual. It appeared to be working as expected, then I received this:
Both CAN and US seem to be connected properly and the traffic data is flowing through....but no revenue/conversion data is flowing. Not sure if you may know why? But I have reached out to google as well.
So I did a little digging. It seems that according to the discussion here, There used to be good integration by shopify for Google Analytics ecommerce, without having to write code. With the upgrade Google Analytics 4, Shopify hasn't integrated with Google Analytics 4 ecommerce yet.
So it looks like we'll have to manually write code to track Google Analytics 4 events. Let's get to some example code!
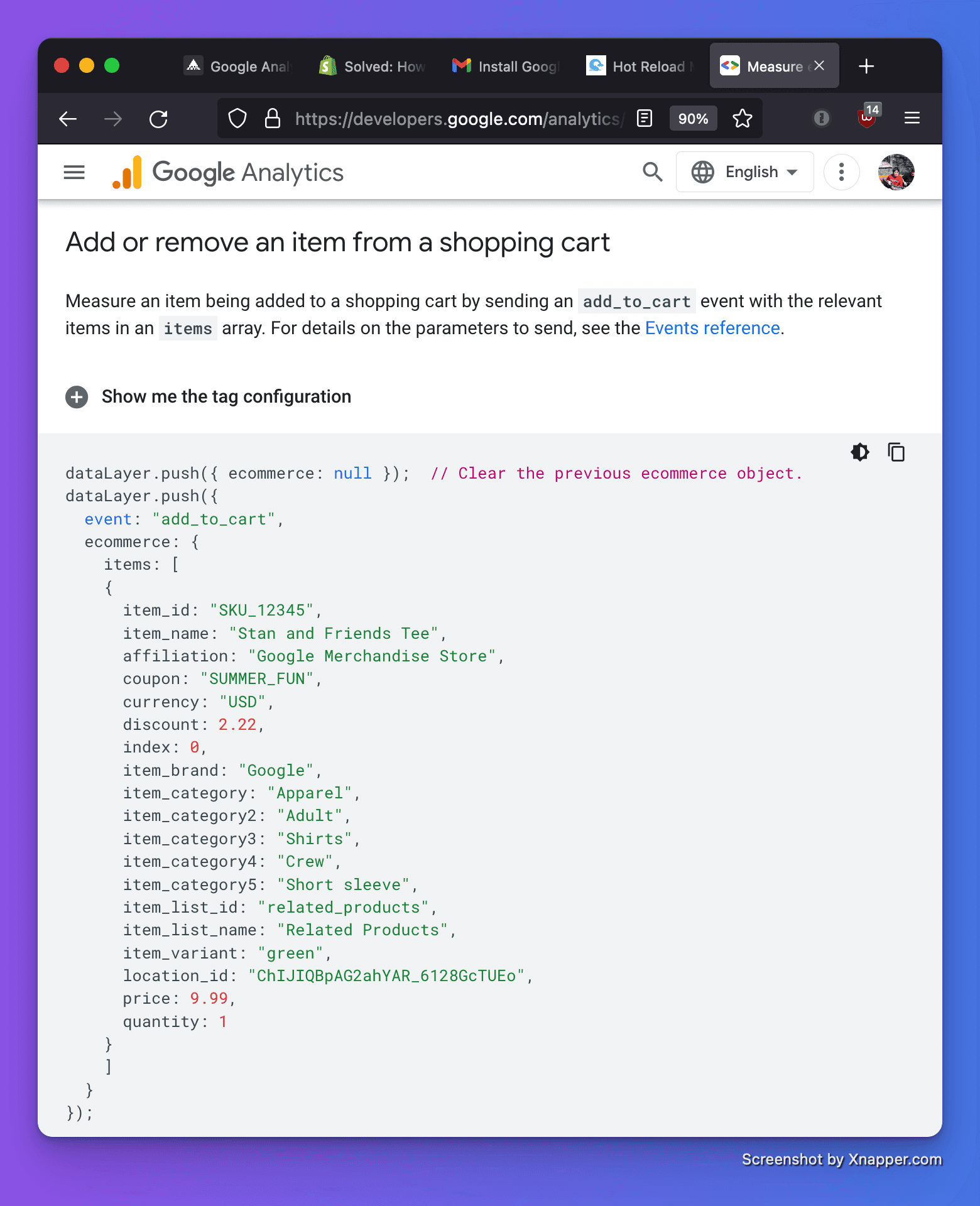
Depending on the theme you're working with, generally adding items to the cart involves submitting a form like this:
<form id="AddToCartForm" method="post" action="/cart/add">
<input type="hidden" name="id" value="{{ product.variants.first.id }}" />
<input min="1" type="number" id="quantity" name="quantity" value="1" />
<input type="submit" value="Add to cart" class="btn" />
</form>
Instead of letting the html form submit using default behavior, we need to run the tracking JavaScript, and ensure that the code finishes before the next page loads.
$(document).ready(() => {
// Attach an add to cart submit event handler
$('body').on('submit', '#AddToCartForm', function (e) {
// prevent default form submission
e.preventDefault();
const $form = $(e.target);
// Run Google Analytics 4 event tracking code here
dataLayer.push({ ecommerce: null });
dataLayer.push(...);
// detach this submit event handler, and proceed with form submission.
$form.off().submit();
});
});
How do we get the dynamic data into the event tracking code, for the item that is actually being added to cart? Let's add data to a hidden input in the form:
<form id="AddToCartForm" method="post" action="/cart/add">
<input type="hidden" name="id" value="{{ product.variants.first.id }}" />
<input
type="hidden"
class="tracking"
name="tracking"
data-title="{{ product.title }}"
data-sku="{{ variant.sku }}"
data-categories="{{ product.collections | map: 'title' | join: ',' }}"
data-brand={{product.vendor | json}}
data-compare={{ product.compare_at_price | money| json }}
data-price={{ product.price_min | money | json }}
data-url="{{ shop.secure_url }}{{ product.url }}"
data-image="https:{{ product.featured_image.src | img_url: 'grande' }}" />
<input min="1" type="number" id="quantity" name="quantity" value="1" />
<input type="submit" value="Add to cart" class="btn" />
</form>
Then we can access the dynamic data attributes from the form, like this:
$(document).ready(() => {
// Attach an add to cart submit event handler
$('body').on('submit', '#AddToCartForm', function (e) {
// prevent default form submission
e.preventDefault();
const $form = $(e.target);
const $trackingInput = $form.find("input.tracking");
const item = {
item_name: $trackingInput.data("title"),
item_id: $trackingInput.data("sku"),
categories: $trackingInput.data("categories").split(","),
price: $trackingInput.data("price"),
...
};
// in item,
// create item_category, item_category2, item_category3,...
// from `data-categories`
$trackingInput
.data("categories")
.split(",")
.forEach((category, ind) => {
item[`item_category${ind == 0 ? "" : ind + 1}`] = category;
});
// pasted from Google Analytics 4 event tracking code example
dataLayer.push({ ecommerce: null });
dataLayer.push({
event: 'add_to_cart',
ecommerce: {
items: [item],
},
});
// detach this submit event handler, and proceed with form submission.
$form.off().submit();
});
});
And we're done with the coding for add-to-cart Ecommerce events with Google Analytics 4! After implementing the code, I used the Google Analytics Debugger to check if it's working. The events are registering in dataLayer as expected:
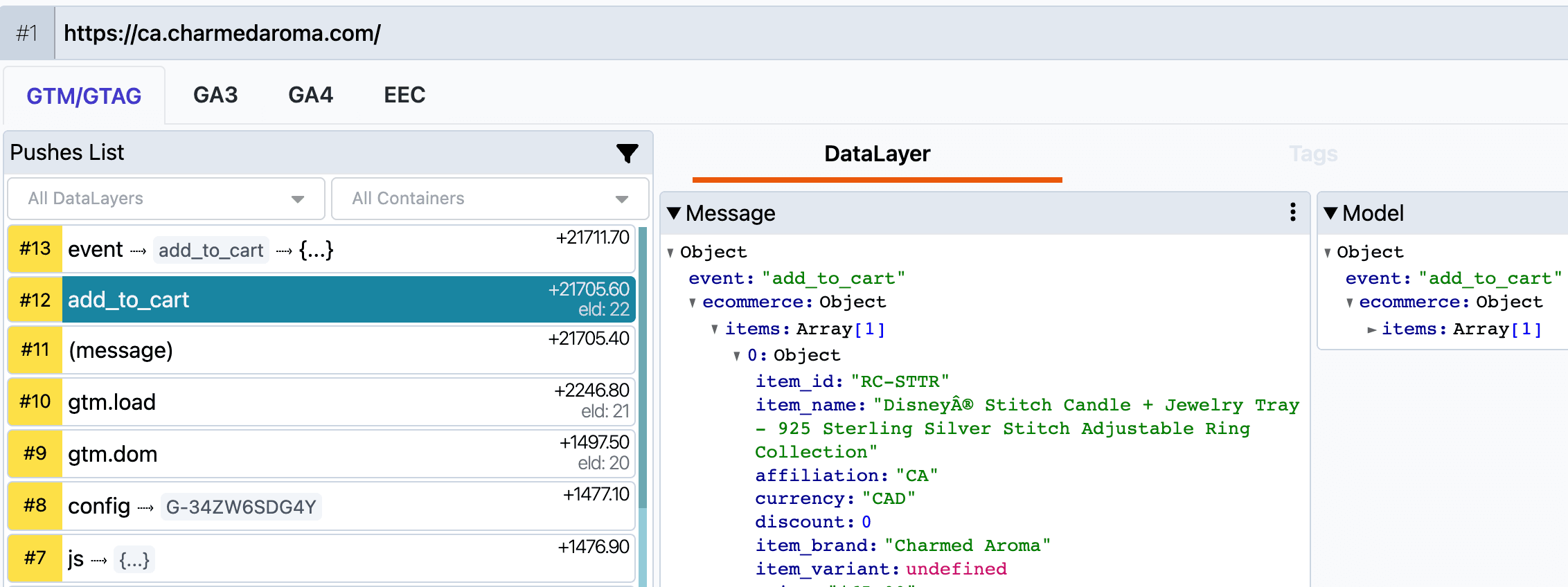
But no add_to_cart
event in GA4. There is only page_view!
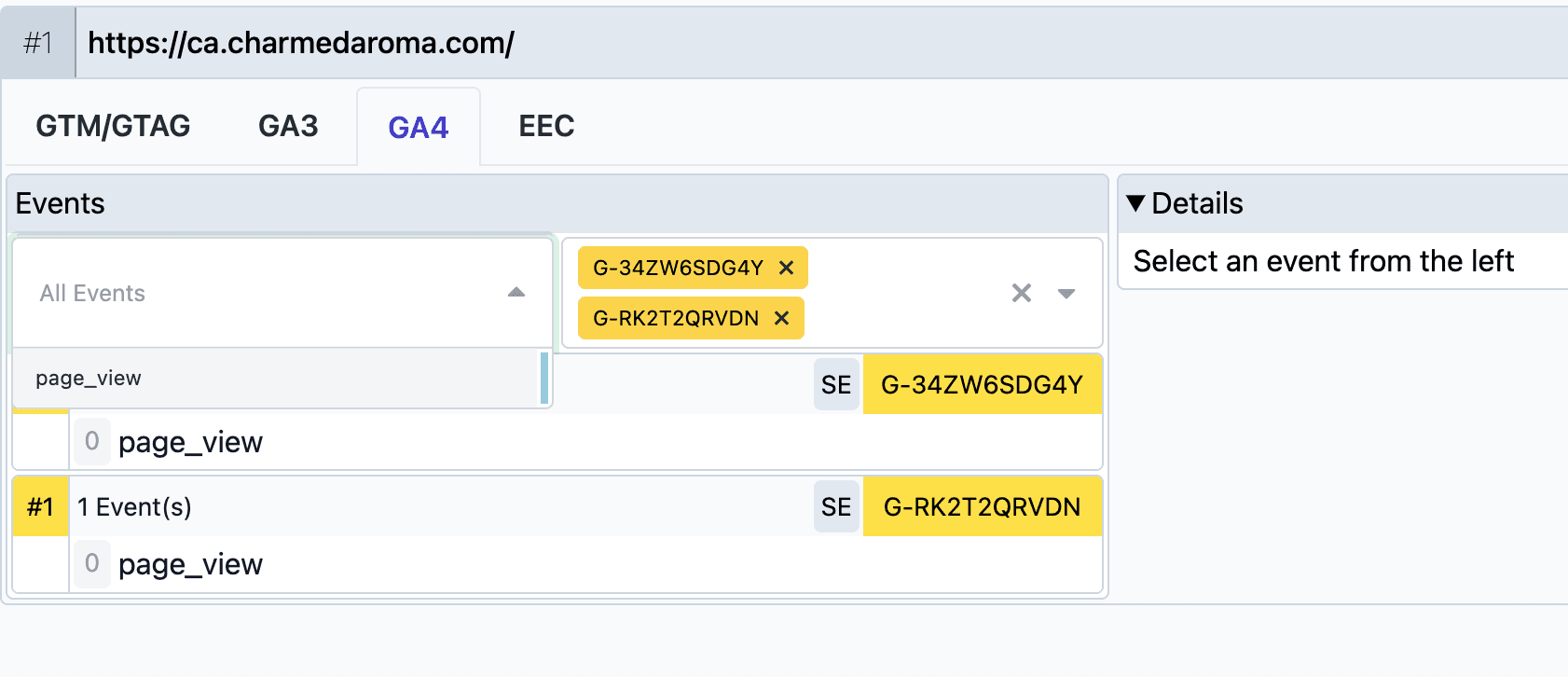
According to GA4's documentation:
To collect ecommerce data, you need to add ecommerce events to your website or app or in your Google Tag Manager container. Because these events require additional context to be meaningful, the events aren't sent automatically
So please make sure that you have the ecommerce events configured properly, else the data won't be collected!
Note that example is not meant for production use, but to provide a general idea of implementing just the add-to-cart event tracking.
Shopify themes tend to be very different. Accurate implementation of event tracking most likely requires skilled dev work. Please feel free to contact us.